|
Overview
of HyperCast
HyperCast
is a software system for self-organizing application-layer overlay
networks. Initially conceived in 1999 for the empirical evaluation of
large-scale reliable multicasting, the software has evolved into a
programming
platform for application-layer overlay networks that accommodates
multiple overlay topology, delivery semantics, support for mobile
networks, authentication and confidentiality, and monitor and control.
HyperCast is implemented in JAVA , though its specification
is language independent.
HyperCast
introduces the concept of an overlay
socket, defined as an
endpoint of communication in an overlay network. An overlay network is
simply viewed as a collection of overlay sockets.
An application program creates an overlay socket,
asks the socket to join an overlay network, an exchange data with other
applications by writing to or reading from the overlay socket. An
overlay socket manage the particiapton of an
applicatoin in an overlay network, such as, discovering neighbors,
maintaining the topology of the overlay networks, and
detecting of partitions.
When an overlay socket is
created, a
configuration file provides, among ohters, information on the overlay
network to join and how to join it, the type of
overlay topology to use, and the type of
substrate network to expect and the addresses to use.
Writing Programs
with Overlay Sockets
Programming
with overlay sockets is similar to network programming with Berkely
sockets. Below is a fragment of a simple application program
that creates an overlay socket. The program reads
a configuration file with name “hypercast.xml” that
contains all information for configuring a overlay network. The overlay
socket joins an overlay network, and then sends the string
“Hello World” to all other overlay sockets in the overlay
network. Then, the application waits for messages sent to the overlay
network and displays each received message.
//This is the
string we want to sent
String
MyString =
new String("Hello World");
//Create an object
that contains configuration parameters
OverlaySocketConfig
ConfObj = OverlaySocketConfig.createConfig("hypercast.xml");
//Create the
overlay socket
I_OverlaySocket
MySocket=ConfObj.createOverlaySocket(null);
//Overlay socket
joins the overlay
MySocket.joinOverlay();
//Create an
application message with “Hello World” payload
I_OverlayMessage
msg =
socket.createMessage(MyString.getBytes(),
MyString.getBytes().length);
//Send the message
to all members in overlay network
MySocket.sendToAll(msg);
//Infinite loop to
receive messages
While(true)
{
//Receive a message from the socket
I_OverlayMessage msg = socket.receive();
//Extract
the
payload
byte[]
data =
msg.getPayload();
//
Print out the
“Hello World” message
System.out.println(“Message
is ” + new String(data) + “.”);
} |
Numerous
utility programs and applications have been developed for HyperCast
overlay sockets, including simple shared applications and a video
streaming system for ad-hoc networks consisting of laptop computers and
PDAs. More complex applications developed or being developed with
HyperCast include an emergency response system and an
information management system for unattended ground
sensors.
Network Topologies
of Hypercast
HyperCast
supports a variety of overlay network topologies. Currently supported
topologies include a spanning tree, a hypercube, a triangulation
graphs, and Pastry, a distributed hash table (DHT) topology. All
overlay topologies
are constructed in a self-organizing fashion. That is, each overlay
socket is responsible for finding and maintaining its neighbors in the
overlay topology. Here we briefly describe two overlay toplogies.
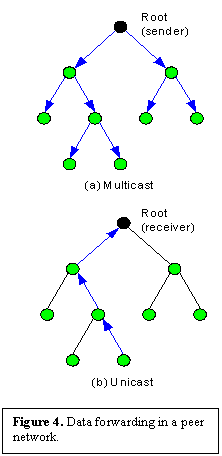
Data transport
In HyperCast, all data is transmitted as formatted
messages trees
that
are dynamically
embedded in an overlay network topology. When an application sends
data to all applications (multicast) in the overlay network, data is
forwarded downstream with the sender as the root of the tree (see
Figure 4(a)). One-to-one (unicast) data is sent upstream in an embedded
tree with the receiver at the root (see Figure 4(b)).
Application data is exchanged in the overlay
network as
formatted
application messages. Each application that participates in a given
overlay network is identified by a logical address specific to the
overlay topology. When an application sends a message to one of its
neighbors in the overlay topology, the message is carried across a
transport-layer network, called the underlay network. One example (but
not the only example) of an underlay network is the public Internet.
When an application message is
transmitted to a neighbor in the overlay network, the logical address
of the neighbor is translated into a address that is recognized by the
underlay network, e.g., an IP address and a port number.
Overlay sockets
HyperCast uses the concept of an overlay socket as an endpoint of
communication in an overlay network. One overlay network comprises
a collection of overlay sockets. When an overlay socket is
created, the socket is configured with a set of attributes that specify
the name of the overlay network to be joined, the type of overlay
protocol and the type of substrate network to be used, as well as
detailed information on the size of internal buffers, protocol-specific
timers, and security properties. Overlay sockets must have compatible
configuration files to join the same overlay network. Each overlay
socket has a logical
address that is dependent on the overlay topology, and a physical
address that specifies an address in the substrate network where
an
overlay socket can send and receive messages. Application programs only
deal with logical addresses, and do not see the physical addresses of
overlay sockets. The software architecture of an overlay socket, shown
in Figure 5, shows the main components of the overlay socket:
- The overlay node
runs a protocol that
establishes and maintains the overlay network topology. The overlay
node is the only component that is aware of the overlay topology.
Eachoverlay topology has its own type of overlay node.
Adding a new overlay topology to the HyperCast software is done by
adding a new type of overlay node and instantiating it in an
overlay socket.
-
The forwarding engine is responsible
for sending, receiving, and forwarding application data in the overlay
network. Application data is transmitted as formatted overlay messages
with logical source
and destination addresses. Overlay messages can be
transmitted to a single overlay socket (unicast) or to all overlay
sockets in the network (multicast).The forwarding engine performs the
functions of an application-level router. It is responsible
for sending, receiving, and forwarding application data in the overlay
network.
-
The message store
is a repository of
transmitted messages that can enhance the delivery service of an
overlay socket, such as services for reliable delivery, resilience of
data, or a data aggregation.
-
Messages are transmitted to neighbors
in the overlay network topologies across a substrate network using
protocols specific to the substrate network. If the substrate network
is Internet-based, then the available protocols in the underlay network
are UDP or TCP. The components in the overlay socket that provide the
interfaces to the substrate network are called adapters. Each overlay
socket has two adapters, a node
adaptor and a socket adapter.
The node
adapter handles the transmission of control messages for maintaining
the overlay network topology and the socket adapter is responsible for
forwarding application data.
An application program interacts with an overlay socket through a set
of interfaces:
-
The overlay
socket application
programming
interface (API) is the main
interface of the overlay socket for sending and receiving applicaiton
data.
-
The statistics API
is used for
monitoring and
managing
internal state information of an overlay socket.
Security architecture
HyperCast includes a security
architecture
for
authentication, and assurance of integrity and confidentiality [ZARI04].
- Authentication:
Authentication is managed through X.509
formatted
certificates signed by a trusted third party or a designated
certificate authority for the overlay network. Overlay sockets
must present certificates when contacting another overlay socket
for the first time.
-
Key
Management:
HyperCast uses a novel neighborhood
key
management scheme where each application shares a key only with
its
immediate neighbors in the overlay network. In this way, changes to the
membership of the overlay network only require the neighbors of a
joining or leaving node to change their keys.
-
Confidentiality
and
Integrity: When an application creates a new message it
uses a message key to ensure integrity and/or confidentiality of the
message. The message key is encrypted with a neighborhood
key, and attached to the message. When the message is forwarded to a
neighbor in the overlay networks, only the message key needs to be
decrypted and encrypted at the neighbor.
HyperCast Applications
The following describes two larger HyperCast applications that were
developed with HyperCast:
-
Location-Based
Messaging for Emergency Response. An analysis of
Arlington County’s emergency response system following the
Pentagon attack in 2001 revealed a lack of situational awareness of
emergency responders. This problem was addressed in a project
with the Arlington County fire department, where we built an awareness
system for emergency responders. A prototype of this system,
implemented with HyperCast, was presented at the Federal Office Systems
Exposition (FOSE) as a Homeland Security Demonstration in Washington,
DC, in April 2003. One component of the system was a messaging system
that gives emergency coordinators the capability to direct messages to
other coordinators and responders based on their geographical
positions. Messages that relate to specific geographic locations are
associated with GPS coordinates and transmitted to overlay networks
assigned to specific geographic regions. Emergency responders equipped
with GPS devices coordinate join overlay networks associated with their
current geographical position. When the responder enters an area
associated with a transmitted message, the message is displayed. For
example, if an emergency coordinator at the Emergency Operations Center
defines a message for a particular city block warning responders of a
fire or riot, responders receive announcements as their GPS receiver
enter the area. To detect whether a responder has entered an area
defined by a message, the software periodically checks the current
position identified by the attached GPS receiver, joins the overlay
that is associated with the current position, and determines whether
this position is within an area defined by a message. The
location-based messaging system permits multiple coordinators (e.g.,
each representing a department, such as fire or police or government
agency) to transmit messages to any authorized responder in a certain
geographic area.
-
Monitoring
Areas. This
scenario is from an ongoing effort
that employs HyperCast to build networks of unattended ground sensors
monitoring small geographical areas or buildings. Each monitored zone
has a small number of passive infrared (PIR), magnetic, and seismic
sensors that trigger imaging sensors (cameras) located in the monitored
zones. Sensors and cameras communicate over an ad-hoc sensor network
using XBow MicaZ motes. Each sensor network has a gateway that can
interface to a mesh radio network built of Novaroam mobile routers or
to an 802.11 network. The goal of the network is to detect personnel
entering or leaving a monitored zone and to take images of
them. The images are sent to different types of users with
different levels of detail. Roaming users equipped with PDAs
approaching the monitored zone can receive information from the sensors
if they are within a specified distance from the zone. In
this case, they receive a summary of the alarm activity in the zone
over the recent past, and all subsequent updates. They can also request
images taken by the imaging sensors within the zone, and can
communicate to the base station to request summaries from sensors in
other monitored areas. Other types of users include analysts that
receive summary updates from all zones at regular intervals.
The updates summarize alarms, images taken, sensor status and mobile
user activity (e.g., entering and exiting the proximity to an area). In
this scenario, there are separate overlays for non-imaging sensor data
and for images transmitted by the camera. For each geographic area
surrounding a monitored zone there is an overlay that mobile users join
as they approach the zone. The underlying network technologies in this
case include sensors/motes that communicate over IEEE 802.15.4
(ZigBee), mesh radios that employ the AODV routing algorithm, and
802.11 wireless local area networks. Some overlay networks cover all of
these technologies.
|
|